Hello, I’m Koha!
1. Basic Concepts
- IO streams handle data transfer between devices
- Java manipulates data through streams
- Java classes for manipulating flows are in the IO package
I/O traffic classification
Byte stream
A byte stream can manipulate any data because any data in a computer is stored in bytes
Example:
1.FileInputStream(using the java.io package) input stream (read)// Create a stream object
FileInputStream fis=new FileInputStream("xxx.txt");
// Reads a byte from the hard disk
int x=fis.read();
System.out.println(x);
int y=fis.read();
System.out.println(y);
int z=fis.read();
System.out.println(z);
// The end tag for reading files is -1
int a=fis.read();
System.out.println(a);
// Close the stream to release resourcesfis.close(); Read all bytes in a file FileInputStream fis=new FileInputStream("xxx.txt");
int t;
while((t=fis.read()) ! = -1){
System.out.println(t);
}
fis.close();
2.FileOutputStream Output (write)// The output stream creates a byte output stream object. If not, a file is automatically created
FileOutputStream fos=new FileOutputStream("yyy.txt");
// Add the value of the AsCll code table
fos.write(97);
fos.write(98);
fos.close();
3.Documents written//FileOutputStream creates an object if it does not have a FileOutputStream. If it does have a FileOutputStream, empty it before writing to it
// The output stream creates a byte output stream object. If not, a file is automatically created
FileOutputStream fos=new FileOutputStream("yyy.txt".true);// If you want to continue, pass the second argument True
// Add the value of the AsCll code table
fos.write(100);
fos.close();
Copy the code
Byte stream buffer
If we pass files byte by byte, it’s inefficient, so we use buffers. BufferedInputStream has a built-in buffer. When a byte is read from BufferedInputStream, BufferedInputStream takes 8192 bytes from the file (2^13), stores them in the buffer, and returns a program, Wait until you fetch the data directly to the buffer until all 8192 bytes have been fetched, and BufferedInputStream will fetch another 8192 bytes from the file.
Directly above:
Here’s an example:
4.Copy image files// Create an input/output stream object
FileInputStream fis=new FileInputStream("copy.png");
FileOutputStream fos=new FileOutputStream("copy2.png");
// Create a buffer object that wraps the input/output stream to make it more powerful
BufferedInputStream bis=new BufferedInputStream(fis);
BufferedOutputStream bos=new BufferedOutputStream(fos);
int b;
while((b=bis.read())! = -1){
bos.write(b);
}
bis.close();
bos.close();
Copy the code
1. Differences between flush and close methods
/ * * *@param args
* @throwsThe IOException * close method flushes the buffer once before closing the stream, flushing all the bytes of the buffer to the file, and closing the stream. * flush * flush * flush */
public static void main(String[] args) throws IOException {
//demo();
// The difference between flush and close methods
BufferedInputStream bis=new BufferedInputStream(new FileInputStream("Copy.png"));
BufferedOutputStream bos=new BufferedOutputStream(new FileOutputStream("Copy3.png"));
int b;
while((b=bis.read())! = -1){
bos.write(b);
}
bos.close();
}
Copy the code
Character stream
Can only operate plain text files, more convenient
Examples of code directly on:
1. Character stream reading
FileReader fr=new FileReader("xxx.txt");
int c;
while((c=fr.read())! = -1) {// Read one character at a time from the project's default code table
char s=(char)c;
System.out.print(s);
}
fr.close();
Copy the code
2. Character stream copy
FileReader fr=new FileReader("xxx.txt");
FileWriter fw=new FileWriter("zzz.txt");
int c;
while((c=fr.read())! = -1){
fw.write(c);
}
fr.close();
fw.close();// The write class has a 2k small buffer that will be written to if the stream is not turned off.! [file](http://image.openwrite.cn/27912_4C25FBE7BD9E4D6688E0F6AE58219354)
Copy the code
Character stream buffer
The BufferedReader class reads text from the character input stream and buffers characters to efficiently read characters, arrays, and lines
Let’s look at a picture:
1. Buffered character stream
/ * * *@param args
* @throwsIOException * special method for buffered streams * readLine() gets a line of text * newLine() function * * difference between newLine()\r\n * newLine() is cross-platform * \r\n Only supports Windows */
public static void main(String[] args) throws IOException {
//demo1();
BufferedReader br=new BufferedReader(new FileReader("xxx.txt"));
BufferedWriter bw=new BufferedWriter(new FileWriter("yyy.txt"));
String line;
while((line=br.readLine())! =null){
bw.write(line);
bw.newLine();// The function of a newline
System.out.println(line);
}
br.close();
bw.close();
}
Copy the code
2. Copy a file to another file in reverse order.
/ * * *@param args
* @throwsIOException * Stream objects open and close as late as possible * * To copy one file to another file in reverse order. * /
public static void main(String[] args) throws IOException {
//1. Create a stream object
BufferedReader br=new BufferedReader(new FileReader("xxx.txt"));
//2. Create a collection object
ArrayList<String> list=new ArrayList<String>();
//3. The read data is stored in the collection
String line;
while((line=br.readLine())! =null){
list.add(line);
}
br.close();
//4. Iterate backwards over the collection data to the file
BufferedWriter bw=new BufferedWriter(new FileWriter("zzz.txt"));
for (int i =list.size(); i >0; i--) {
bw.write(list.get(i));
bw.newLine();
}
/ / 5. Turn off the flow
bw.close();
}
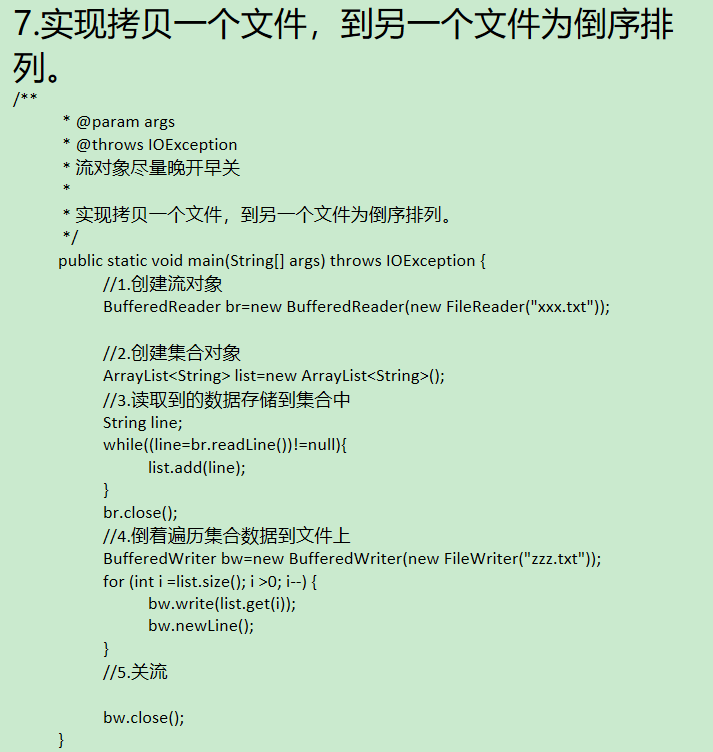
Copy the code
5. Other flows
1.SequenceInputStream (combining two input streams into one
FileInputStream fis1=new FileInputStream("a.txt");
FileInputStream fis2=new FileInputStream("b.txt");
SequenceInputStream sis=new SequenceInputStream(fis1,fis2);
FileOutputStream fos=new FileOutputStream("c.txt");
int b1;
while((b1=sis.read())! = -1) {// keep reading bytes from a.txt
// Read bytes are written to c.txt
fos.write(b1);
}
sis.close();// When sis is closed, all stream objects passed in by the constructor are also closed
fos.close();
Copy the code
2.ByteArrayOutputStream Memory output stream
FileInputStream fis=new FileInputStream("a.txt");
// Create a memory array that can grow in memory
ByteArrayOutputStream bos=new ByteArrayOutputStream();
int b;
while((b=fis.read())! = -1){
bos.write(b);
}
// Get all the data in the buffer and assign it to the arR array
byte[] arr=bos.toByteArray();
System.out.println(new String(arr));
// Converts the contents of the buffer to a string. Adjustments can be omitted in the output statement.
System.out.println(bos.toString());
fis.close();
Copy the code
3. Random access stream RandomAccessFile
The RandomAccessFile class is not a stream. It is a subclass of Object, combining InputStream and OutputStream, and can read and write files randomly.
RandomAccessFile raf=new RandomAccessFile("g.txt"."rw"); //rw is read and write permission
raf.seek(10);// Set the pointer at the specified position
raf.write(97);
raf.close();
Copy the code
4. The flow of the operation object
The stream reads an object, or writes it to a program, and performs serialization (archiving) and deserialization (reading).
Example:1.ObjectOutputStream ObjectOutputStream serialization (relative to archive) Person p1=new Person("Zhang".23);
Person p2=new Person("Bill".23);
ObjectOutputStream oos=new ObjectOutputStream(new FileOutputStream("a.txt"));
oos.writeObject(p1);
oos.writeObject(p2);
2.ObjectInputStream deserialization (equivalent to reading files) ObjectInputStream ois=new ObjectInputStream(new FileInputStream("a.txt"));
Person p1=(Person)ois.readObject();
Person p2=(Person)ois.readObject();
System.out.println(p1);
System.out.println(p2);
ois.close();
Copy the code
Full object Write, Read (Optimized)
3.Full object Write, Read (Optimized)/ / write
Person p1=new Person("Zhang".23);
Person p2=new Person("Bill".23);
Person p3=new Person("Fifty".23);
ArrayList<Person> list=new ArrayList<Person>();
list.add(p1);
list.add(p2);
list.add(p3);
ObjectOutputStream oos=new ObjectOutputStream(new FileOutputStream("a.txt"));
oos.writeObject(list);
oos.close();
/ / read
ObjectInputStream ois=new ObjectInputStream(new FileInputStream("a.txt"));
ArrayList<Person> arrlist=(ArrayList<Person>)ois.readObject();
for (Person person : arrlist) {
System.out.println(person);
}
ois.close();
Copy the code